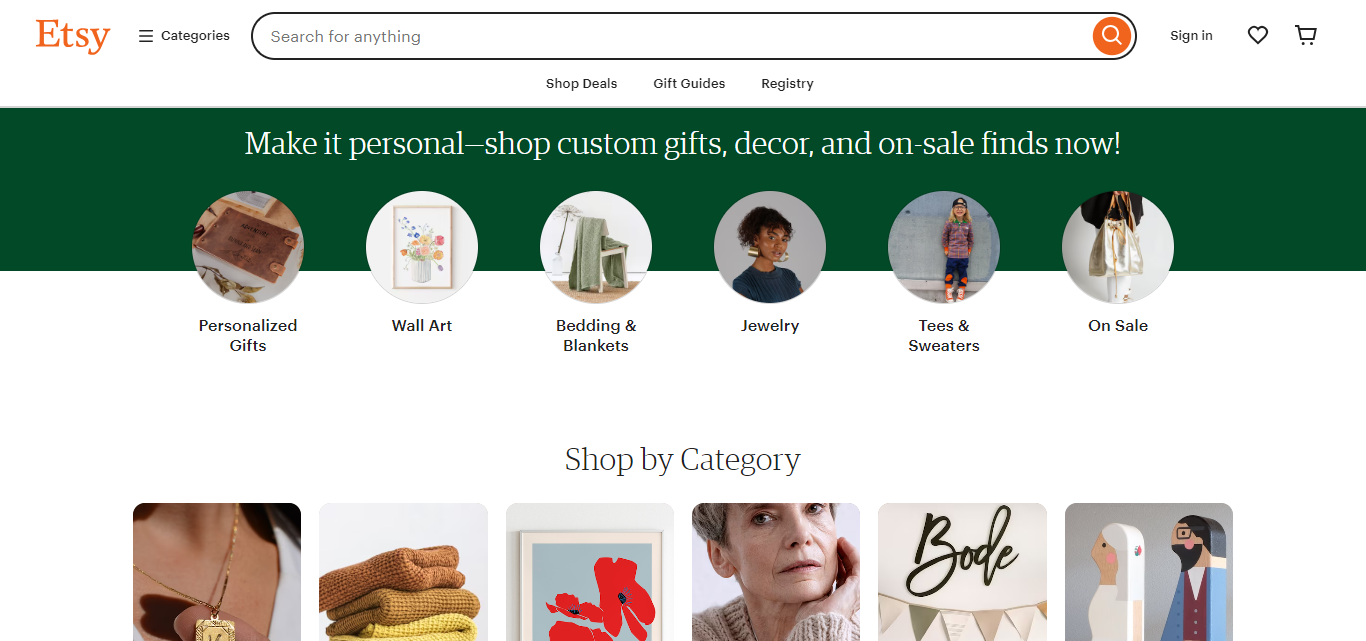
Help me create an etsy api integration.
Softnir! To create an Etsy API integration, you'll need to go through several steps to ensure you can access Etsy's API and communicate with it from your application. Here's a general step-by-step guide:
Create an Etsy Developer Account:
- Sign up for an Etsy developer account at the Etsy Developer site (https://www.etsy.com/developers/).
- Register your application to receive an API key. This key will be used in all API requests.
Read the Documentation:
- Familiarize yourself with Etsy’s API documentation (https://www.etsy.com/developers/documentation).
- Understand the API limits, terms of use, and the data you can access.
Identify Your Needs:
- Determine the functionality you want to implement with the Etsy API (e.g., accessing shop data, managing listings, processing sales).
Set Up Your Development Environment:
- Choose your programming language and set up your development environment.
- Make sure you have all relevant SDKs, libraries, or frameworks required to make HTTP requests to the Etsy API.
Authenticate with OAuth:
- Implement OAuth authentication as required by Etsy to allow your application secured access to an Etsy account.
- This will generally involve redirecting the user to Etsy for authorization and receiving an access token in response.
Develop Your Application:
- Begin coding your integration using the chosen programming language.
- Implement the necessary API endpoints to perform actions like getting shop information, modifying listings, or handling transactions.
- Use your API key and adhere to Etsy API standards and naming conventions.
Testing:
- Test your API calls with a sandbox or test environment, if Etsy provides one.
- Make sure your application handles different types of responses, including success, errors, and edge cases.
Error Handling:
- Ensure your application gracefully handles API errors and limitations.
- Implement logging for debugging and operational purposes.
Review Etsy’s Terms and Conditions:
- Make sure your application complies with Etsy’s terms of use for API developers.
- Be aware of rate limits and conduct proper rate handling to avoid being blocked.
Deploy Your Integration:
- After thorough testing, deploy your Etsy API integration.
- Monitor the integration’s performance and make adjustments as required.
Keep Up with API Changes:
- Regularly check for any updates to Etsy’s API and update your application as necessary to maintain functionality.
Is there a specific part of this process where you would like more detailed assistance or clarification? Also, if you have questions about implementation in a specific programming language or environment, feel free to ask!